- October 17th, 2021
- Code
- By Steve Shead
A simple notifications script that you can adapt to your needs
Updated on January 13th, 2022 by Steve Shead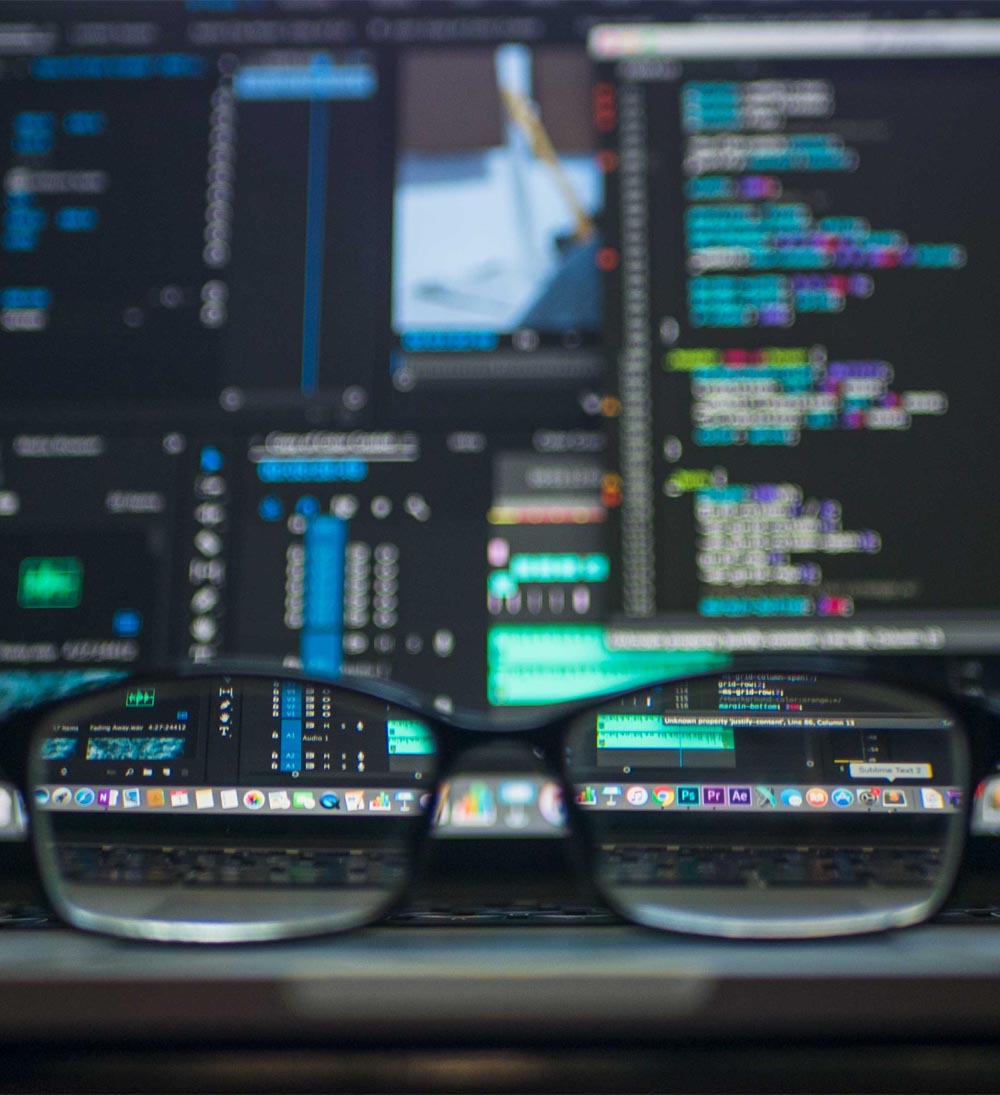
- October 17th, 2021
- Code
- By Steve Shead
- 0
- 0
A simple notifications script that you can adapt to your needs
I thought I would share the basic notifications script I used in the backend of this website. What I'm about to walk through is not what I used, it is what I started with and adapted for my use. In the admin dashboard admins can create notifications, alerts and comments for other admins to see. I also adapted this script such that the system can send automated alerts when a new user or a new comment is added (for instance). I spent a fair amount of time adjusting and rewriting for my needs - you should probably do the same.
Note that if you use this script you'll at the very least need to sanitize inputs and will want to setup the database connection more robustly than has been done here - and probably do some more cleanup. I'm giving the framework, the basics - you can adapt to your needs.
I found a script on the web and converted it to Boostrap 5, as well as cleaning it up a bit for my use. I'm sharing what I found but as noted above it will still need some work. You might not like they way it is layed out, and that's fine. Adapt it for your use. The good thing is it does work as is. As I continue to develop this site I'll add the ability to download files, but, right now it's going to be code on a page.
Here's what it looks like. The first is the form where you input the notification:
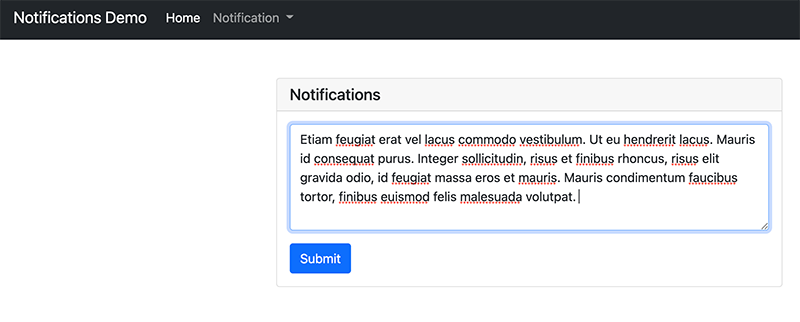
The second shows the notification icon in the navbar, and the notification itself in the dropdown.
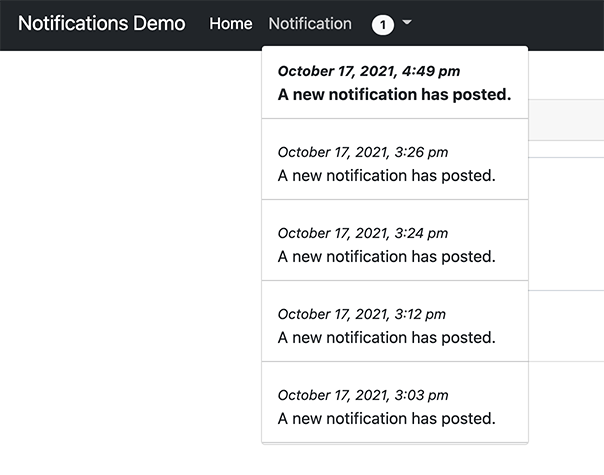
And the third shows the notification on a page, selected from the database.
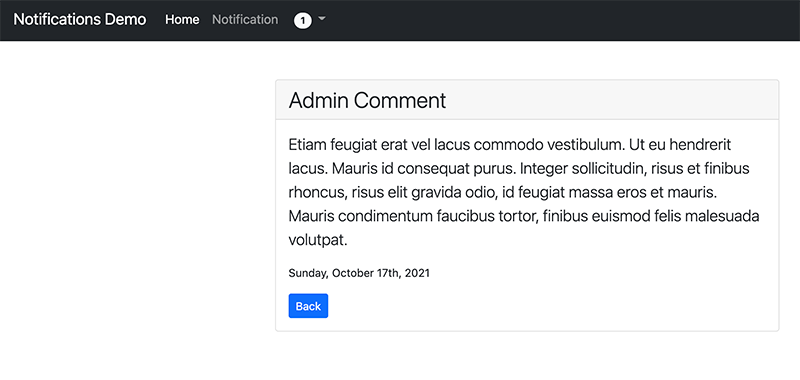
Let's get started.
You'll need to create a database, or have a database you want to use. I called the table 'notifications' in mine to keep it simple. Here's the database sql file. Create a file called notifications.sql and paste the code below into it, then import it into your database - it will create the required table and populate some data. Remember to make sure you edit the constants.php file with your database connection details.
--
-- Table structure for table `notifications`
--
CREATE TABLE `notifications` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` text NOT NULL,
`type` text NOT NULL,
`message` text NOT NULL,
`status` text NOT NULL,
`date` datetime NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- Dumping data for table `notifications`
--
INSERT INTO `notifications` (`id`, `name`, `type`, `message`, `status`, `date`) VALUES
(1, 'Steve', 'comment', 'This is the first notification', 'unread', '2021-10-17 00:21:21'),
(2, 'Joe', 'comment', 'This is the second notification', 'unread', '2021-10-17 00:22:21'),
(3, 'Jane', 'comment', 'This is the second notification', 'unread', '2021-10-17 00:23:21');
Next, in the root directory where you want the notifications script to live, create the two files below.
-- index.php
-- view.php
Here's the code for index.php:
<?php
include 'includes/functions.php';
include 'includes/header.php';
include 'includes/navbar.php';
if (isset($_POST['submit'])) {
$message = $_POST['message'];
$query = "INSERT INTO notifications (id, name, type, message, status, date) VALUES (NULL, '', 'comment', '$message', 'unread', CURRENT_TIMESTAMP)";
if (performQuery($query)) {
header("location: index.php");
}
}
?>
<div class="container my-5">
<div class="row">
<div class="col-lg-6 mx-auto">
<div class="card">
<div class="card-header">
<h5 class="mb-0">Notifications</h5>
</div>
<div class="card-body">
<form method="post" class="form-inline my-2 my-lg-0">
<textarea name="message" class="form-control me-sm-2 mb-3" placeholder="Notification" rows="5" required></textarea>
<button name="submit" class="btn btn-primary my-2 my-sm-0" type="submit">Submit</button>
</form>
</div>
</div>
</div>
</div>
</div>
<?php include 'includes/footer.php'; ?>
and here's the code for view.php:
<?php
include 'includes/functions.php';
include 'includes/header.php';
include 'includes/navbar.php';
$id = $_GET['id'];
$query ="UPDATE notifications SET status = 'read' WHERE id = '$id';";
performQuery($query);
?>
<div class="container my-5">
<div class="row">
<div class="col-lg-6 mx-auto">
<div class="card">
<div class="card-header">
<h3 class='fw-light mb-0'>Admin Comment</h3>
</div>
<div class="card-body">
<?php
$query = "SELECT * from notifications WHERE id = '$id';";
if(count(fetchAll($query)) > 0){
foreach(fetchAll($query) as $i){
$date = date("l, F jS, Y", strtotime($i['date']));
echo "<p class='lead'>" . $i['message'] . "</p>";
echo "<p class='small'>" . $date . "</p>";
}
}
?>
<a class="btn btn-sm btn-primary" href="index.php">Back</a>
</div>
</div>
</div>
</div>
</div>
<?php include 'includes/footer.php'; ?>
Now create a folder called 'includes', and create the five files below in that folder.
-- constants.php
-- footer.php
-- functions.php
-- header.php
-- navbar.php
Here's the code for each file.
constants.php
<?php
define('DBINFO', 'mysql:host=localhost;dbname=notifications');
define('DBUSER','root');
define('DBPASS','');
footer.php
<!-- Bootstrap Bundle with Popper -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.0/dist/js/bootstrap.bundle.min.js" integrity="sha384-U1DAWAznBHeqEIlVSCgzq+c9gqGAJn5c/t99JyeKa9xxaYpSvHU5awsuZVVFIhvj" crossorigin="anonymous"></script>
</body>
</html>
functions.php
<?php include 'constants.php';
function fetchAll($query){
$con = new PDO(DBINFO, DBUSER, DBPASS);
$stmt = $con->query($query);
return $stmt->fetchAll();
}
function performQuery($query){
$con = new PDO(DBINFO, DBUSER, DBPASS);
$stmt = $con->prepare($query);
if($stmt->execute()){
return true;
} else {
return false;
}
}
header.php
<?php ob_start(); ?>
<!doctype html>
<html lang="en">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- Bootstrap CSS -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.0/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-KyZXEAg3QhqLMpG8r+8fhAXLRk2vvoC2f3B09zVXn8CA5QIVfZOJ3BCsw2P0p/We" crossorigin="anonymous">
<title>Notifications System</title>
<style>
.scrollable-menu {
height: auto;
max-height: 400px;
overflow-x: hidden;
}
</style>
</head>
<body>
navbar.php
<nav class="navbar navbar-expand-lg navbar-dark bg-dark">
<div class="container">
<a class="navbar-brand" href="#">Notifications Demo</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSupportedContent">
<ul class="navbar-nav me-auto mb-2 mb-lg-0">
<li class="nav-item">
<a class="nav-link active" aria-current="page" href="#">Home</a>
</li>
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" href="#" id="navbarDropdown" role="button" data-bs-toggle="dropdown" aria-expanded="false">Notification
<?php
$query = "SELECT * FROM notifications WHERE status = 'unread' ORDER BY `date` DESC";
if(count(fetchAll($query))>0){
?>
<span class="badge bg-light text-dark rounded-circle ms-3"><?php echo count(fetchAll($query)); ?></span>
<?php
}
?>
</a>
<ul class="dropdown-menu scrollable-menu" aria-labelledby="navbarDropdown">
<?php
$query = "SELECT * FROM notifications ORDER BY date DESC";
if(count(fetchAll($query)) > 0){
foreach(fetchAll($query) as $i){
?>
<a style ="
<?php
if($i['status'] == 'unread'){
echo "font-weight:bold;";
}
?>
" class="dropdown-item" href="view.php?id=<?php echo $i['id'] ?>">
<small><i><?php echo date('F j, Y, g:i a',strtotime($i['date'])) ?></i></small><br/>
<?php echo "A new notification has posted."; ?>
</a>
<li><hr class="dropdown-divider"></li>
<li>
<a class="dropdown-item" href="#">
<?php }
} else {
echo "No Records yet.";
}
?>
</a>
</li>
</ul>
</li>
</ul>
</div>
</div>
</nav>
Now you have the structure and code for this to work. Assuming you've done this right it should work as is. Note the notification alert will appear in the menu bar next to the word "notification", and the new notification will appear in the dropdown menu.
There is a whole lot more than this than just copying and pasting code. There are nuances in the code that some might not like, and that is ok. In the spirit of learning, finding issues is a part of the learning curve - and securing code a must. That being said, if there is something totally insecure I'd really like to know. Whilst I didn't use this code as is, I still used this as the foundation of what I built.
As always, let me know your thoughts and ideas, good or bad, either way. I'm happy to listen and learn.
Happy coding!
Cheers
~Steve
Delete Post?
Are you sure you want to delete this post? This action cannot be undone!
Husband, father, grandfather, C Suite leader, engineer, designer, photographer, videographer, musician, composer, pilot, geek, daring to be different - yeah, busy!
All author posts